class JDBCDemo {
private String driver="com.mysql.jdbc.Driver"
;
private String url="jdbc:mysql://localhost:3306/test"
;
private String username="root"
;
private String password="root"
;
Connection conn=
null;
Statement statement=
null;
ResultSet set=
null;
public void updateSql(){
try{
//加载驱动类
Class.forName(driver);
//与数据库建立连接
conn=
DriverManager.getConnection(url,username,password);
//发送SQL语句
statement=
conn.createStatement();
//插入数据
String sql="insert into user(username,password) values(‘114‘,‘1234‘)"
;
//修改数据
//String sql="update user set password=‘1232‘ where id=2"
//删除数据
//String sql= "delete from user where id=2";
//sql语句结果,一般进行 增删改 的SQL语句用excuteUpdate
int count=
statement.executeUpdate(sql);
if(count>0
){
System.out.println("操作成功"
);
}else{
System.out.println("操作失败"
);
}
}catch(ClassNotFoundException e){
e.printStackTrace();
}catch (SQLException e){
e.printStackTrace();
}catch (Exception e){
e.printStackTrace();
}finally {
try {
if (statement ==
null) {
//用判断来防止statement空异常
statement.close();
}
if (conn ==
null) {
conn.close();
}
}catch (SQLException e){
e.printStackTrace();
}
}
}
public void querySql(){
try{
//加载驱动类
Class.forName(driver);
//与数据库建立连接
conn=
DriverManager.getConnection(url,username,password);
//发送SQL语句
statement=
conn.createStatement();
String sql="select * from user where username=‘111‘ and password=‘1234‘ "
;
//返回结果集
set=
statement.executeQuery(sql);
while(set.next()){
//用两种方式获取值,一种是根据列名,一种是所在的列数
String name=set.getString("username"
);
String pass=set.getString("password"
);
//String name=set.getString(2);
// String pass=set.getString(3);
System.out.println(name+","+
pass);
}
}catch(ClassNotFoundException e){
e.printStackTrace();
}catch (SQLException e){
e.printStackTrace();
}catch (Exception e){
e.printStackTrace();
}finally {
try {
if (statement ==
null) {
//用判断来防止statement空异常
statement.close();
}
if (conn ==
null) {
conn.close();
}
}catch (SQLException e){
e.printStackTrace();
}
}
}
上述代码主要使用Statement方式发送SQL,还有preparedStatement方式。他们之间有相同点,但是又不是一样,下面就来比较下Statement和PreparedStatement
二、Statement与PreparedStatement的比较
Statement:(1)产生方式:Connection.createStatement()(2)增删改 executeUpdate()(3)查询 executeQuery()(4)一般将SQL语句放在executeUpdate()中
PreparedStatement:(1)产生方式:Connection.prepareStatement()(2)增删改 executeUpdate()(3)查询 executeQuery()(4)一系列SetXXX方法(5)将SQL放在Connection.PrepareStatement(SQL)(6)需要预编译
查看PreparedStatement的源码 ,可知PreparedStatement继承Statement,Statement里有的方法PreparedStatement都有,但是PreparedStatement还有很多SetXXX方法

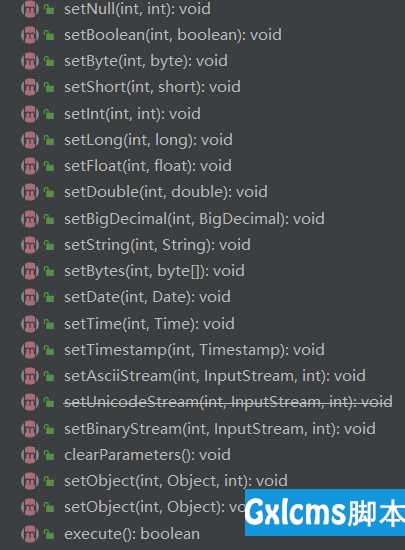
用代码直观的体现两者的区别:
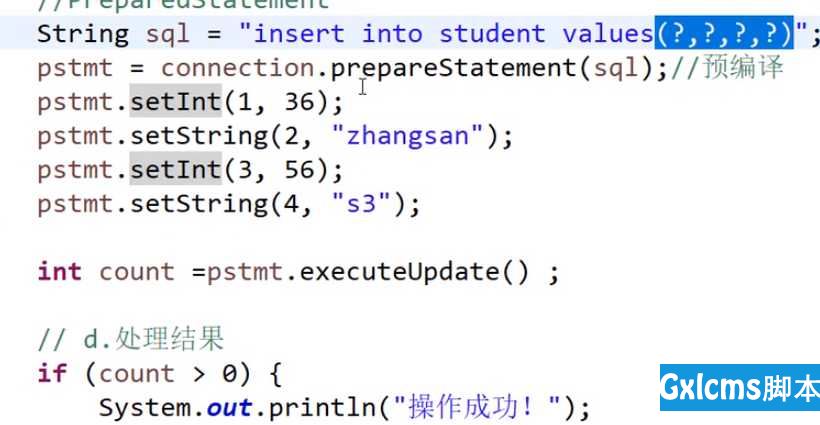
除了这部分之外,其他和上述代码一样,多了一步预编译。
三、PreparedStatement较Statement的优势
(1)编码更加简洁灵活:在PreparedStatement中SQL语句后的查询条件,插入的值可以用占位符(?)来表示,不用像Statement一样写具体的值,只需要调用PreparedStatement中的SetXXX方法赋值即可。
(2)提高性能:如果要执行一条SQL语句100回,Statement需要connection,createStatement(sql)语句编译SQL100次,PreparedStatemnet只需要编译SQL语句一回(即connection.PreparedStatement(sql)),重复执行100次preparedStatement.executeQuery();减少编译SQL次数,提高性能。
(3)安全性高,可以有效防止SQL注入的风险:Statement存在SQL注入的风险,PreparedStatement可以防止SQL注入的风险。
java篇之JDBC原理和使用方法
标签:delete 获取 ext 不用 条件 jdbc原理 col sof upd